Magic of Increment and Decrement Operators
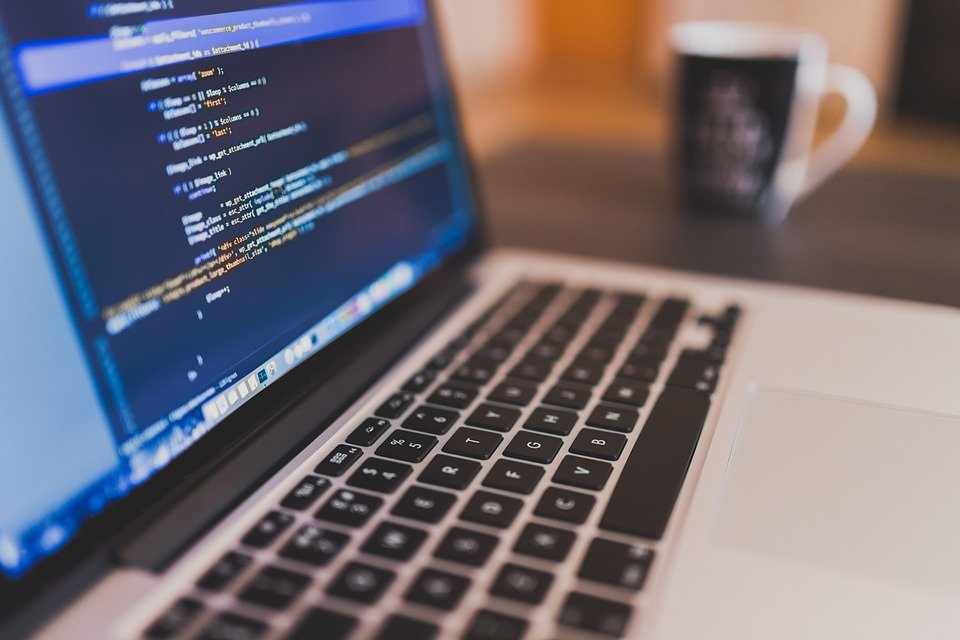
I have spent a lot of time trying to understand the true funda of increment\decrement operators during my graduation. During campus recruitment there was always at least one question that asked for the output of an expression that consisted a mixture of increment and decrement operators. Everyone knows these operators increment or decrement the value of a variable by 1.
++i is a pre-increment operator
i++ is a post-increment operator
–i is a pre-decrement operator
i– is a post-decrement operator
These are quite clear since they are bare basics. Here I am sharing a strategy I have adopted to review code (specifically java code) and find the final value without running any code.
Increment operators in an expression
Now let us consider how to understand how the operators will behave in an expression. Let us start with simplest of all first. Study the code below.
int i = 0; int j = 0; i = i++; j = ++j;
The value of i and j will be 0 and 1 respectively. Let us now take a little more difficult example. Consider the following code.
int i = 0; i = i++ + ++i + ++i;
Now this is quite difficult to evaluate. Now let us try to see how we can figure out the final value of i without executing the code. I have a small technique of mine (probably adopted by many other java gurus out there long time back). I first go from left to right in the expression (after the assignment operator). Only a small list of rules:
- Replace the pre operators with the modified value.
- Replace the post operators with the un-modified value.
- Keep track of the modification happened to the variable.
Now let us take the code and evaluate it. The blue color is a marker to see the progress.
Expression | Value of i |
---|---|
i = i++ + ++i + ++i; | 0 |
i = 0 + ++i + ++i; | 1 |
i = 0 + 2 + ++i; | 2 |
i = 0 + 2 + 3; | 3 |
Final Value = “0 + 2 + 3″ | 5 |
The modified value will be the value of i mentioned in the same row and the unmodified value will be the value of i in the previous row.
Note: In case there are more than one variable associated, add new columns to track those additional variables.
Note: This strategy might not be correct in case of other programming languages. In most cases, this will do fine.
Let us try the same for the following code.
int i = 0; i = i++ + i++ + ++i + ++i + i++ + i++;
Now the evaluation goes like this:
Expression | Value of i |
---|---|
i = i++ + i++ + ++i + ++i + i++ + i++; | 0 |
i = 0 + i++ + ++i + ++i + i++ + i++; | 1 |
i = 0 + 1 + ++i + ++i + i++ + i++; | 2 |
i = 0 + 1 + 3 + ++i + i++ + i++; | 3 |
i = 0 + 1 + 3 + 4 + i++ + i++; | 4 |
i = 0 + 1 + 3 + 4 + 4 + i++; | 5 |
i = 0 + 1 + 3 + 4 + 4 + 5; | 6 |
Final Value = “0 + 1 + 3 + 4 + 4 + 5″ | 17 |
Decrement operators in an expression
We can apply the same rules on the decrement operator also. Let’s lake a simple example.
int i = 10; i = --i + i-- + --i;
Now let’s analyze the above code.
Expression | Value of i |
---|---|
i = –i + i– + –i; | 10 |
i = 9 + i– + –i; | 9 |
i = 9 + 9 + –i; | 8 |
i = 9 + 9 + 7; | 7 |
Final Value = “9 + 9 + 7″ | 25 |
That’s cool, isn’t it.
More complex expressions
We can use the same strategy for more complex expressions. Consider the following code.
int i = 5; i = --i - i++ + ++i * --i * i-- + i++;
Let’s evaluate this code.
Expression | Value of i |
---|---|
i = –i – i++ + ++i * –i * i– + i++; | 5 |
i = 4 – i++ + ++i * –i * i– + i++; | 4 |
i = 4 – 4 + ++i * –i * i– + i++; | 5 |
i = 4 – 4 + 6 * –i * i– + i++; | 6 |
i = 4 – 4 + 6 * 5 * i– + i++; | 5 |
i = 4 – 4 + 6 * 5 * 5 + i++; | 4 |
i = 4 – 4 + 6 * 5 * 5 + 4; | 5 |
Final Value = “4 – 4 + 6 * 5 * 5 + 4″ | 154 |
Multiple variables in an expression
Now let us see how to handle multiple variables. Consider the following code.
int i = 5; int j = 10; i = --i + ++j * --i * j-- + i++ - ++j;
As I have mentioned earlier, there will be separate columns for tracking values of i and j.
Expression | Value of i | Value of j |
---|---|---|
i = –i + ++j * –i * j– + i++ – ++j; | 5 | 10 |
i = 4 + ++j * –i * j– + i++ – ++j; | 4 | 10 |
i = 4 + 11 * –i * j– + i++ – ++j; | 4 | 11 |
i = 4 + 11 * 3 * j– + i++ – ++j; | 3 | 11 |
i = 4 + 11 * 3 * 11 + i++ – ++j; | 3 | 10 |
i = 4 + 11 * 3 * 11 + 3 – ++j; | 4 | 10 |
i = 4 + 11 * 3 * 11 + 3 – 11; | 4 | 11 |
Final Value = “4 + 11 * 3 * 11 + 3 – 11″ | 359 | 11 |
Try Yourself
Now try some of these expressions, and of course in your spare time
Consider initial value of i and j as 25 and 10 respectively.
i = i++ * ++i / –i * i++ * i++ + –i * –i; i = ++i / ++i * i++ * –i * –i + i++ * i–; i = ++i * ++i * i++ * –i; i = ++i + ++j + i++ / –j * j++; i = ++i + ++j + j++ + –i * ++j + j–;